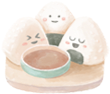
click
blue text above
Follow us~
Inheritance can be said to be one of the important features of object-oriented, it can reduce the amount of code duplication. Inheritance can also make the code clearer. Inheriting or overriding the parent class method makes it more convenient to maintain the code and write the code more flexibly. Inheritance can be understood as the inheritance of nature, which is the process in which a subclass acquires property from the parent class; therefore, inheritance in programming is the process in which the subclass acquires member variables and member functions from the parent class .
Python's inheritance is public inheritance, not as complicated as C++, and the inheritance syntax is also very simple:
class 子类名(父类名):
子类类体
Among them, the parent class is also called the base class, super class ; the child class is also called the derived class .
Example 1 : Inheritance
class People(object):
def __init__(self, name):
self.name = name
def say(self):
print("我是{},是一个人".format(self.name))
class Student(People):
def say(self):
print("我是{},是一个学生".format(self.name))
Among them, People is the parent class and Sudent is the subclass. The base class of People is object . Note that it is the root base class of all classes in Python 3.
Student inherits all the methods and properties of the base class People, so both name and __init__ can be used. At the same time, Student also rewrites the say method, which will override the say method in People. See example:
stu = Student("范进")
stu.say()
p = People("严监生")
p.say()
Output result:
我是范进,是一个学生
我是严监生,是一个人
Inheritance in Python has the following properties:
-
Members that are not in the subclass will be automatically searched in the superclass. For example, the initialization of Student will use Pe o ple 's __inint__
-
Private members cannot be inherited and accessed, but are still placed in the __dict__ dictionary
-
All inheritance is public inheritance
-
Both subclasses and instances can freely access public members; private members are hidden and cannot be accessed directly
-
The private variable can be accessed in the method in the class where the private variable is located
-
The attribute search process is from instance dict-->class dict-->parent class dict, in turn to find
Example 2 : Verify the above properties
stu = Student("范进")
stu.say()
p = People("宋江")
print(stu.name)
# print(stu.__age) # 私有属性不可访问
# stu.__get_age() # 私有方法不可访问
print("{}".format(People.__dict__))
print("{}".format(Student.__dict__))
In addition, you can use the following magic properties to get some parameters:
-
__base__ : the base class of the class
-
__based__ : tuple of base classes for the class
-
__mro__ : Display method lookup order, tuple of base classes
-
__subclasses__() : a list of subclasses of the class
-
mro(): Display method lookup order, tuple of base classes
Example 3 : Test the above properties
print("{}".format(People.__dict__))
print("{}".format(Student.__dict__))
print(stu.__dict__)
print(stu.__class__.mro())
print(Student.__base__)
print(People.__subclasses__())
print(Student.__bases__)
print(Student.__mro__)
print(Student.mro())
output:
It can be seen that Student inherits from People, and People inherits from object.
The last is the initialization of inheritance. The __init__ method is not defined in the Student class above, and it will automatically use this method of People for initialization. If the subclass wants to define an __init__ method by itself, then this method will override the method of the parent class, so that the function of the parent class cannot be used normally. To solve this problem, the super keyword needs to be used.
Example 4 : Using the super keyword
class Student(People):
def __init__(self, name, weight):
super(Student, self).__init__(name)
self.weight = weight
def say(self):
print("我是{},是一个学生, 体重是{}".format(self.name, self.weight))
The super keyword is used in line 4. super (Student, self ) means to find the parent class of Student , and then use the method of this parent class to pass in the corresponding parameters.
Instantiate an object, then call the say method:
stu = Student("范进", 68)
stu.say()
output:
我是范进,是一个学生, 体重是68

point to share
Like
click to see