1. SQLite interface
1.1 open
SQLITE_API int sqlite3_open(
const char *filename, /* Database filename (UTF-8) */
sqlite3 **ppDb /* OUT: SQLite db handle */
);
1.2 exec
SQLITE_API int sqlite3_exec(
sqlite3*, /* An open database */
const char *sql, /* SQL to be evaluated */
int (*callback)(void*,int,char**,char**), /* Callback function */
void *, /* 1st argument to callback */
char **errmsg /* Error msg written here */
);
1.3 close
SQLITE_API int sqlite3_close(sqlite3*);
2. The callback function in exec
This article mainly talks about the exec interface and callback in SQLite. Let's first look at the parameters in exec:
-
sqlite3* The handle returned by the database opened by the open function;
-
const char *sql is the SQL statement;
-
int(*callback)(void*,int,char**,char**) callback function, usually used to receive data queried by SQL statement;
-
void *, this parameter is used as the first parameter in the callback function;
-
char **errmsg If there is an error during execution, it will be written here.
int callback(void* data, int argc, char** argv, char** azColName)
For example I now have a database as shown below:
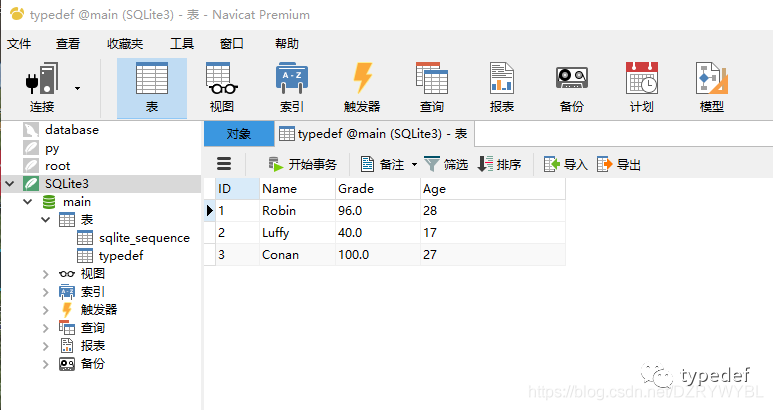
First of all, the callback does not just call the callback function once exec is executed, but it is determined according to the result of the query. If the result satisfying the SQL statement has 100 rows, the callback function will be called 100 times, and each callback function is used to obtain a row of records, which can also be seen in the following test routines.
As shown in the table above, I want to query the record whose ID is 1. The first parameter of the callback function is the parameter passed in by the user, that is, the fourth parameter of the exec function, and the second parameter is how many fields are in the query result. (column), the number is 4 in the above table, the third parameter is the value of the field, namely (1, Robin, 96.0, 28), and the fourth parameter is the field name, namely (ID, Name, Grade, Age ), just one row of data can be obtained through these three parameters. Then, the obtained data is passed out through the first parameter.
3. Routine code
The following code is to query all the records in the above chart and print the data.
#include <stdio.h>
#include <stdlib.h>
#include "sqlite3.h"
static int callback(void* data, int argc, char** argv, char** azColName) {
int i;
fprintf(stderr, "%s: ", (const char*)data);
for (i = 0; i < argc; i++) {
printf("%s = %s\n", azColName[i], argv[i] ? argv[i] : "NULL");
}
printf("\n");
return 0;
}
int main(int argc, char* argv[]) {
sqlite3* db;
char* zErrMsg = 0;
int rc;
char* sql;
const char* data = "Callback function called";
/* Open database */
rc = sqlite3_open("typedef.db", &db);
if (rc) {
fprintf(stderr, "Can't open database: %s\n", sqlite3_errmsg(db));
exit(0);
} else {
fprintf(stderr, "Opened database successfully\n");
}
/* Create SQL statement */
sql = "SELECT * from typedef";
/* Execute SQL statement */
rc = sqlite3_exec(db, sql, callback, (void*)data, &zErrMsg);
if (rc != SQLITE_OK) {
fprintf(stderr, "SQL error: %s\n", zErrMsg);
sqlite3_free(zErrMsg);
} else {
fprintf(stdout, "Operation done successfully\n");
}
sqlite3_close(db);
return 0;
}
The execution result is as follows:
Due to the recent changes in the push rules of the WeChat public platform, many readers reported that they did not see the updated articles in time. According to the latest rules, it is recommended to click "recommended reading, sharing, collection", etc. to become frequent readers.
Recommended reading:
Please click [Watching] to add chicken legs to the editor
